목록알고리즘 (29)
\(@^0^@)/
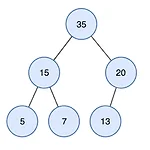
이진 힙(Binary Heap) 은 이진트리 형식을 취하는 힙 데이터 구조입니다. 바이너리 힙은 우선순위 대기열을 구현하는 일반적인 방법입니다. 상위 키가 하위 키보다 크거나 같은(≥) 힙을 최대 힙이라고 합니다. 작거나 같은 것을 최소 힙이라고 합니다. https://en.wikipedia.org/wiki/Binary_heap Binary heap - Wikipedia From Wikipedia, the free encyclopedia Jump to navigation Jump to search Variant of heap data structure Binary (min) heapTypebinary tree/heapInvented1964Invented byJ. W. J. WilliamsAlgorithm..
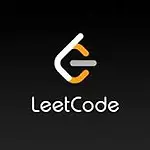
Reverse Words in a String III Input: s = "Let's take LeetCode contest" Output: "s'teL ekat edoCteeL tsetnoc" Input: s = "God Ding" Output: "doG gniD" 어려운 문제는 아닌데 지난번에 풀지 못하여서 오늘 재도전하였다. 살짝 야매?로 푼 것 같긴 한데... 그래도 제출되었으니 성공 :) 내가 푼 코드는 이렇다. var reverseWords = function(s) { if(s === "") return -1 let splited = s.split(" ") let result = []; let store; for(let i = 0; i < splited.length; i++) { store =..
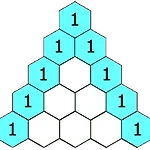
Pascal's Triangle II https://leetcode.com/explore/learn/card/array-and-string/204/conclusion/1171/ Explore - LeetCode LeetCode Explore is the best place for everyone to start practicing and learning on LeetCode. No matter if you are a beginner or a master, there are always new topics waiting for you to explore. leetcode.com Input: rowIndex = 3 Output: [1,3,3,1] Input: rowIndex = 0 Output: [1] 내가..
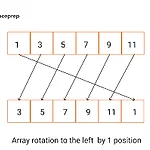
rotate array https://leetcode.com/explore/learn/card/array-and-string/204/conclusion/1182/ Explore - LeetCode LeetCode Explore is the best place for everyone to start practicing and learning on LeetCode. No matter if you are a beginner or a master, there are always new topics waiting for you to explore. leetcode.com 🔅 내가 시도한 코드 내가 푼 코드는 이렇다. 콘솔에서는 잘 찍히지만 leetcode에 제출하면 어떻게 된 영문인지, 위의 이미지와 같이 [5,..
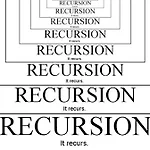
stringifyNumbers : 모든 values들의 numbers를 strings으로 변환하는 문제. 내 출력 값이 제공받은 것과 같게 나왔지만 오답이었다.... /* 내가 작성한 코드 */ function stringifyNumbers(obj) { for (let key in obj) { if (typeof obj[key] === "object") { stringifyNumbers(obj[key]); } else if (typeof obj[key] === "number") { JSON.stringify(obj[key]); } } return obj; } 콘솔상으로는 nested object 안의 모든 숫자들을 string으로 변환한 것 같은데, 채점을 돌려보면 그렇지 않은 모양이다ㅠ 분명 1에서 '..
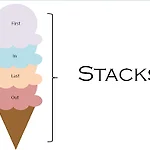
스택 (Stack) 나중에 넣은 데이터가 먼저 나오는 LIFO(Last In First Out) 기반의 선형 자료 구조 구현 메서드 (method) 데이터 전체 획득 / 비어 있는지 확인 : Stack.getBuffer(), Stack.isEmpty() 추가 / 삭제 / 마지막 데이터 조회 / 크기 확인 : Stack.push(), Stack.pop(), Stack.peak(), Stack.size() 데이터 위치 / 존재 여부 확인 : Stack.indexOf(), Stack.includes() Stack() : 생성자 함수로 초기 데이터 설정 getBuffer() : 객체 내 데이터 셋 반환 isEmpty() : 객체 내 데이터 존재 여부 파악 // Stack(): 생성자 함수로 초기 데이터 설정 f..
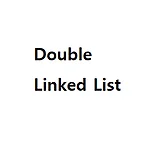
이중 연결 리스트 (Double Linked List) 각 노드가 데이터와 포인터를 가지며, 두 줄로 연결되어 있는 방식으로 데이터를 저장하는 자료 구조 구현 메서드 (method) 노드 개수 / 비어 있는지 확인 : DoubleLinkedList.size(), DoubleLinkedList.isEmpty() 순차 출력 / 역 출력 : DoubleLinkedList.printNode(), DoubleLinkedList.printNodeInverse() 노드 추가 : DoubleLinkedList.append(), DoubleLinkedList.insert() 노드 삭제 : DoubleLinkedList.remove(), DoubleLinkedList.removeAt() 데이터 위치 확인 : DoubleL..
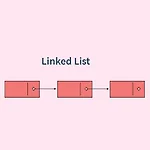
TDL에 정리하기에는 너무 내용이 길어서, 보기 쉽게 한 번에 정리하기 위하여 TIL로 작성. Linked-list를 1부터 6까지 다양한 구현 메서드를 활용해보자! 연결 리스트 (Linked List) 각 노드가 데이터와 포인터를 가지며, 한 줄로 연결되어 있는 방식으로 데이터를 저장하는 자료 구조 구현 메서드(method) 노드 개수/ 비어 있는지 확인/ 노드 출력 : LinkedList.size(), LinkedList.isEmpty(), LinkedList,printNode() 노드 추가 : LinkedList.append(), LinkedList.insert() 노드 삭제 : LinkedList.remove(), LinkedList.removeAt() 데이터 위치 확인 : LinkedList.i..
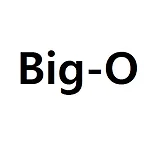
- Objectives - 1. Motivate the need for something like Big O Notation Big O Notation과 같은 것에 대한 동기 부여 2. Describe what Big O Notation is / Big O 표기법이 무엇인지 설명 3. Simplify Big O Expressions / Big O 표현식 단순화 4. Define "time complexity" and "space complexity" / "시간 복잡도"와 "공간 복잡도" 정의 5. Evaluate the time complexity and space complexity of different algorithms usgin Big O Notation 시간 복잡도를 평가하고 Big O 표기법..
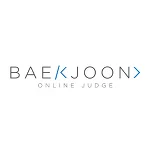
🐱👓 1. 문제 : 1152 https://www.acmicpc.net/problem/1152 🔥 2-1. 코드 및 풀이 (실패) ㅇ처음에는 너무 쉽게 생각해서 이런 식으로 구현하였는데, 첫 번째 예제에 바로 먹히길래 제출하였는데 당연히 탈락. const fs = require('fs'); const file = process.platform === 'linux' ? '/dev/stdin' : './input.txt'; let input = fs.readFileSync(file).toString().split("\n"); let words = input.join(",").split(" "); // The Curious Case of Benjamin Button console.log(words); // ..